Summary: in this tutorial, you’ll learn about C# extension methods and how to use them to extend any type with additional methods.
Introduction to C# extension methods
To add a method to a class, we often subclass the existing class and add the method to the derived class.
C# introduced a new feature called extension methods that allows you to add new methods to an existing type (or class) without the need of using inheritance.
An extension method is a static method but it is called as if it was an instance method on the extended type.
Creating C# extension methods
To create an extension method, you define a static class that contains the extension method. For example, the following creates an extension method on the string
type:
using System.Globalization;
static class StringExtensions
{
public static string ToTitleCase(this string value)
{
return CultureInfo.CurrentCulture.TextInfo.ToTitleCase(value);
}
}
Code language: JavaScript (javascript)
How it works.
- First, define the static class called
StringExtensions
. - Second, define the extension method
ToTitleCase()
as a static method of theStringExtensions
class. The extension method usesthis
keyword before the first parameter. In this example, theToTitleCase()
method uses the current culture’s text info to convert a string to a title case.
Using C# extension methods
The following program demonstrates how to call the ToTitleCase()
extension method on a string:
using System.Globalization;
static class StringExtensions
{
public static string ToTitleCase(this string value)
{
return CultureInfo.CurrentCulture.TextInfo.ToTitleCase(value);
}
}
class Program
{
public static void Main(string[] args)
{
var message = "C# extension methods are cool";
var result = message.ToTitleCase();
Console.WriteLine(result);
}
}
Code language: JavaScript (javascript)
Output:
C# Extension Methods Are Cool
Code language: PHP (php)
A more practical example of the C# extension method
The following example demonstrates how to use extension methods to extend the int type by adding two methods IsEven()
and IsOdd()
:
static class IntExtensions
{
public static bool IsEven(this int value)
{
return value % 2 == 0;
}
public static bool IsOdd(this int value)
{
return !IsEven(value);
}
}
class Program
{
public static void Main(string[] args)
{
int[] numbers = { 3, 5, 6, 2, 7, 8 };
foreach (var number in numbers)
{
if (number.IsEven())
{
Console.WriteLine(number);
}
}
}
}
Code language: PHP (php)
Output:
6
2
8
In this example, the IsEven()
method returns true
if the number is even and false
otherwise. The IsOdd()
method is the reverse of the IsEven()
method.
In the Main()
method of the Program
class, we define a list of integers, iterate over its elements using a foreach, and write the even number to the console. We use the IsEven()
extension method to check if a number is even or not.
Advantages of C# extension methods
Extension methods have the following advantages:
- Allow you to add functionality to the types that you don’t control such as .NET classes without the need of subclassing them.
- Make your code cleaner and more readable by keeping related methods in one place.
- Better discoverability: When working with an object, you can find all its extension methods via IntelliSense.
The following picture shows the available extension methods (IsEven
& IsOdd
) of the integer type that we defined in the previous example:
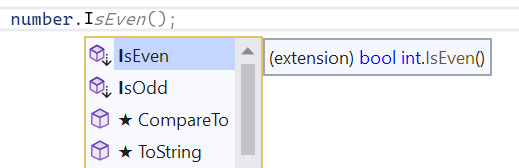
Summary
- C# extension methods allow you to extend an existing type without using inheritance.