Summary: in this tutorial, you’ll learn about C# raw string literals that have been introduced in C# 11.
Introduction to the C# raw strings
C# string literals require escaping of special characters and delimiters. Therefore, it’s difficult to construct literal strings specially for regexes as well as XML and JSON. For example:
var path = "C:\temp\no.txt";
Console.WriteLine(path);
Code language: JavaScript (javascript)
In this example, the \t
means tab and \n
means new line. It outputs the following to the console:
C: emp
o.txt
Code language: CSS (css)
To fix it, you need to use a verbatim string by prefixing the string with the @
character:
var path = @"C:\temp\no.txt";
Console.WriteLine(path);
Code language: JavaScript (javascript)
Also, if a string contains quotes "
, you need to escape it using the \
characters. For example:
var response = "{ \"name\": \"John Doe\"}";
Console.WriteLine(response);
Code language: JavaScript (javascript)
The string becomes difficult to read.
C# 11 introduced raw string to overcome the problems. A raw string uses a flexible start and end delimiter to avoid conflict with the contents of the string.
A raw string starts with a minimum of three quotes """
and is optionally followed by a new line, the content of the string, and ends with the same number of quotes.
For example:
var json = """{ "name": "John Doe"}""";
Console.WriteLine(json);
Code language: JavaScript (javascript)
Output:
{ "name": "John Doe"}
Code language: JSON / JSON with Comments (json)
If the string itself may use three quotes then the raw string can have longer starting/ending delimiters, which are four quotes like this:
var s = """" C# raw string uses a minimum of """ """";
Console.WriteLine(s);
Code language: PHP (php)
Output:
C# raw string uses a minimum of """
Code language: PHP (php)
Multi-line raw string literals
C# raw string literals support multiline strings. For example:
var json = """
{
"name": "John Doe",
"address": {
"home": "4000 N. 1st street",
"work": "101 N. 1st street"
}
}
""";
Console.WriteLine(json);
Code language: PHP (php)
Output:
{
"name": "John Doe",
"address": {
"home": "4000 N. 1st street",
"work": "101 N. 1st street"
}
}
Code language: JSON / JSON with Comments (json)
The multi-line raw string literals follow these rules:
- The opening and closing quotes of a multi-line raw string literal must be on separate lines.
- Any whitespace following the opening quote on the same line is ignored.
- Whitespace-only lines following the opening quotes are included in the string literal.
- Any whitespace to the left of the closing quotes is removed from all lines of the raw string literal.
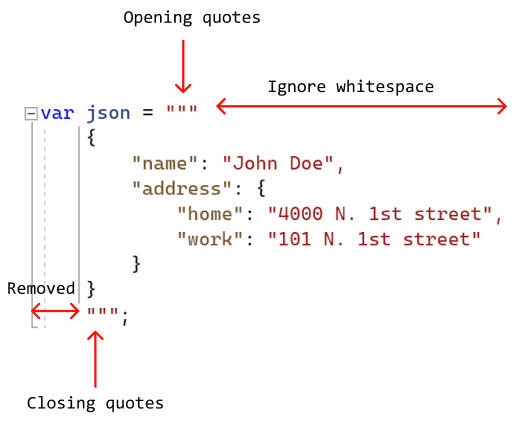
Raw string interpolation
Raw string literals also support string interpolation that replaces the variables and expressions inside the string with their values.
The following example causes an error because of unexpected {}
in JSON format:
var name = "John Doe";
var json = $""" {"Name": "{name}" } """;
Console.WriteLine(json);
Code language: JavaScript (javascript)
To fix this, you need to surround the variable with double opening and closing curly braces {{}}
. Also, you need to use double $$
characters:
var name = "John Doe";
var json = $$""" { "Name": "{{name}}" } """;
Console.WriteLine(json);
Code language: JavaScript (javascript)
Output:
{ "Name": "John Doe" }
Code language: JSON / JSON with Comments (json)
Summary
- Use a minimum of opening and closing
"""
to form a raw string literal. - C# raw string literals support multi-line strings and interpolations.