Summary: in this tutorial, you’ll learn how to rethrow an exception in C# using the throw
statement.
When to rethrow exceptions in C#
In C#, you can use the try...catch
block to catch an exception in the try
block and handle it accordingly in the catch
block.
In some cases, you may want to re-throw an exception after you have caught it in the catch
block.
This can be useful if you need to log the exception first and then propagate it up the call stack, which you will handle in another catch
block.
The following illustrates how to rethrow an exception:
try
{
// Some code that might throw an exception
}
catch (Exception ex)
{
// Handle the exception
Console.WriteLine($"An exception occurred: {ex.Message}");
// Rethrow the exception
throw;
}
Code language: C# (cs)
In this syntax, the code in the try
block might throw an exception. If an exception occurs, we handle it in the catch
block.
In the catch
block, we handle the exception by showing a message to the console and use rethrow it using the throw
statement.
C# rethrow exceptions example
The following example demonstrates how to rethrow an exception using the throw
statement:
static float Divide(int a, int b)
{
return a / b;
}
try
{
var result = Divide(10, 0);
}
catch (DivideByZeroException ex)
{
Console.WriteLine(ex.Message);
// rethrow the exception
throw;
}
Code language: C# (cs)
How it works.
First, define the Divide()
method that returns the division of two integers.
Second, divide 10 by zero using the Divide
method. In the catch block, we log the exception message to the console and rethrow the same DivideByZeroException
exception.
Catching and rethrowing another exception
Sometimes, you want to handle an exception and wrap it in a new exception, and propagate the new exception up the call stack.
To do that you use the throw
statement with the new exception and you pass the original exception to the inner exception parameter of the new exception. For example:
static float Divide(int a, int b)
{
return a / b;
}
try
{
var result = Divide(10, 0);
}
catch (DivideByZeroException ex)
{
Console.WriteLine(ex.Message);
// rethrow the exception
throw new ArithmeticException(ex.Message, ex);
}
Code language: C# (cs)
In this example, we log the message of the DivideByZeroException
exception to the console.
Also, we wrap the
into a new DivideByZeroException
exception by assigning the ArithmeticException
exception to the DivideByZeroException
InnerException
property of the
exception.ArithmeticException
The following shows the ArithmeticException
with DivisionByZeroException
as the inner exception:
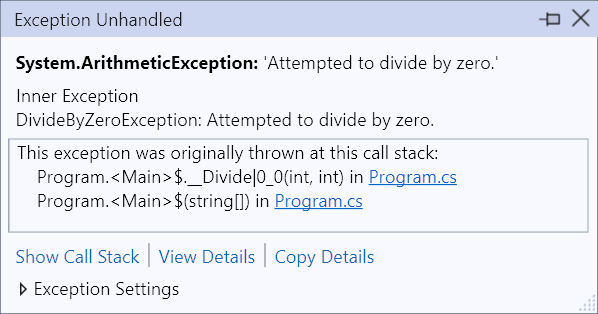
Summary
- Rethrow an exception when you want to do something like logging before propagating the same exception up the call stack.
- Use the
throw
statement to rethrow the same exception.