Summary: in this tutorial, you’ll learn how to create a simple program that displays the famous message Hello, World! on a console window.
Creating the C# hello world program
First, launch Visual Studio.
Next, click the Create a New Project button:
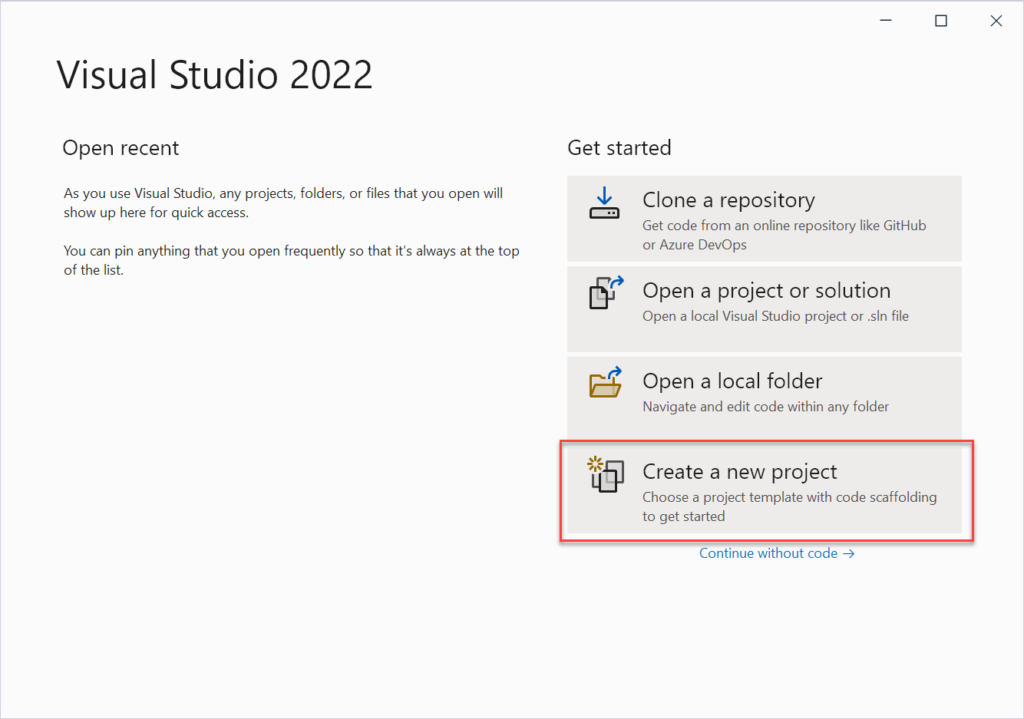
Then, select the Console App to create a project for a command-line application that runs on .NET core on Windows, Linux, and macOS, and click the Next button:
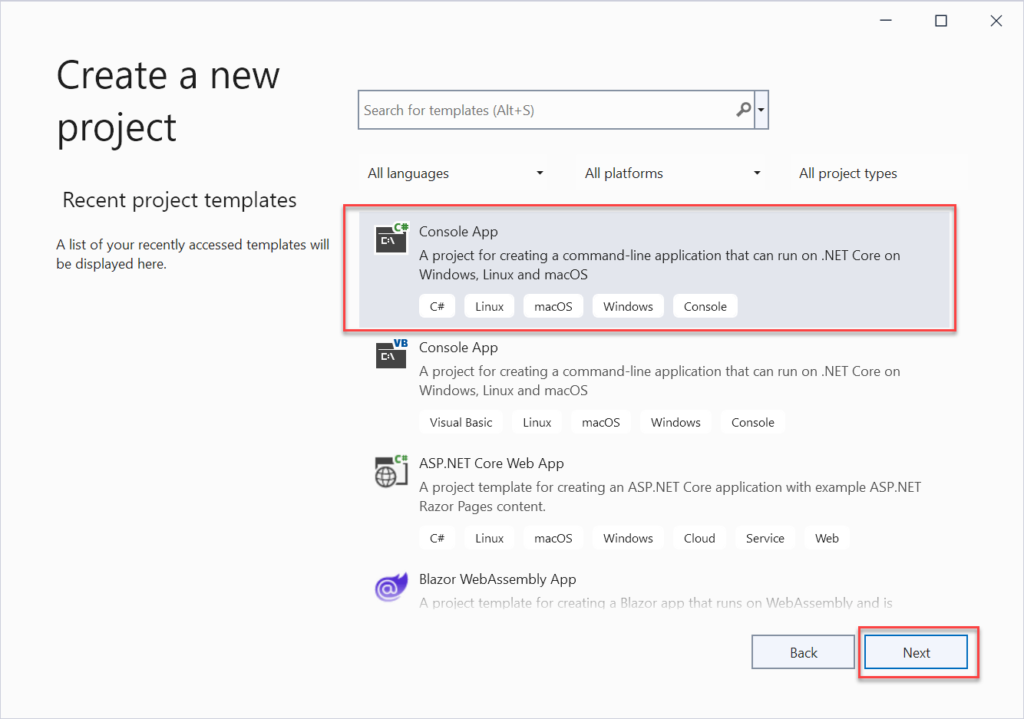
After that, configure the new project by entering the project name, location, and solution name, and click the Next button:
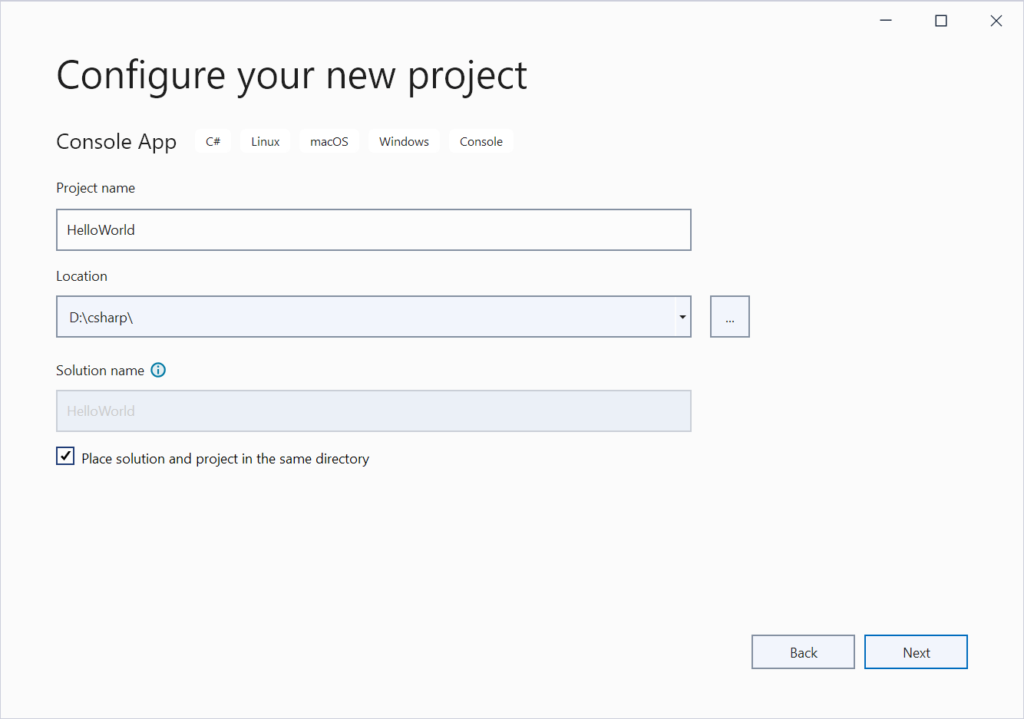
Finally, select the .NET framework, 6.0 (Long-term support) in this case and click the Create button:
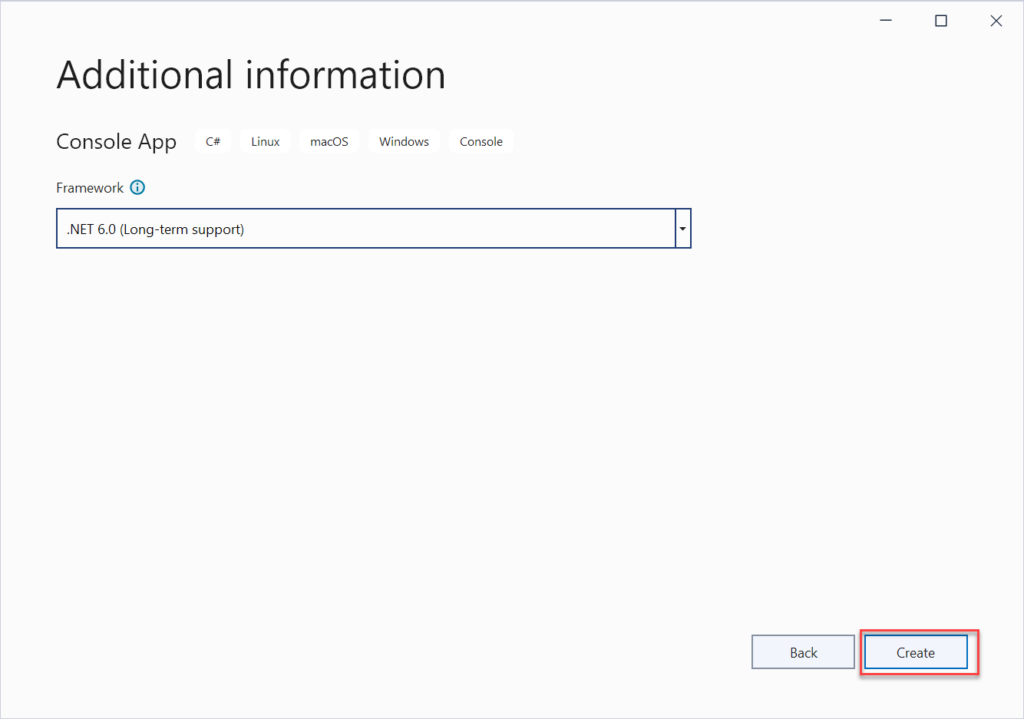
The Visual Studio will show up as follows:
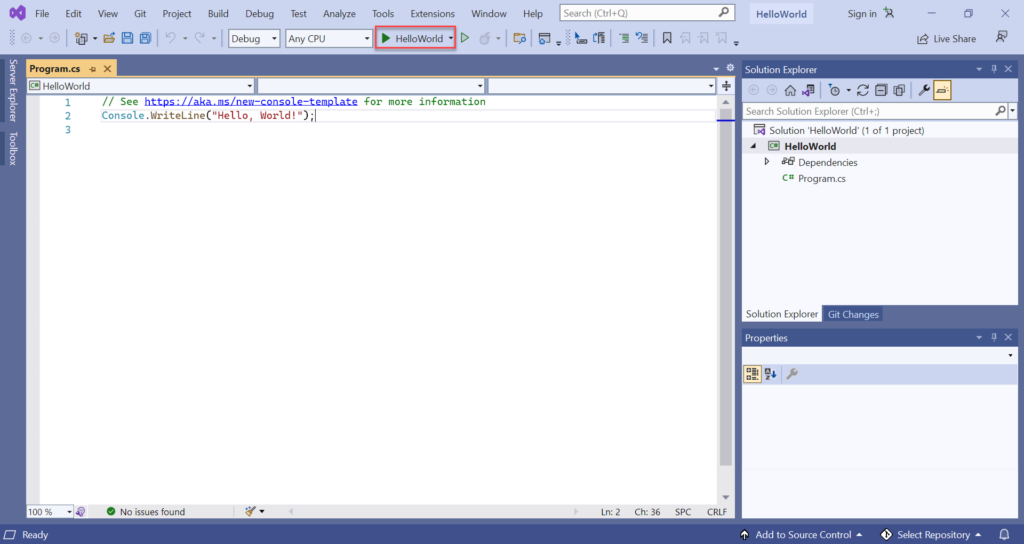
On the left-hand side is the code editor where you can enter the C# code. By default, Visual Studio automatically generates a statement that shows the Hello, World!
message.
Also, Visual Studio shows the solution explorer on the right pane. A solution may contain one or more projects. In this example, the HelloWorld
solution has one project with the same name.
Under the HelloWorld
project is the source code file program.cs
. Note that all C# source code files have the extension .cs
that stands for CSharp.
The following shows the contents of the program.cs file:
// See https://aka.ms/new-console-template for more information
Console.WriteLine("Hello, World!");
Code language: C# (cs)
In this program:
The first line that starts with double forward slashes //
is a comment. It doesn’t have any effect on the program:
// See https://aka.ms/new-console-template for more information
Code language: C# (cs)
The second line writes the message "Hello, World!"
to the console:
Console.WriteLine("Hello, World!");
Code language: C# (cs)
In this code, the Console.WriteLine
is a method that accepts a text as the input and writes that text to the console window.
To run the program, you click the HelloWorld
button or press the F5 keyboard. Behind the scene, VS will compile and run the program. The following console window will display the “Hello, World!” message:
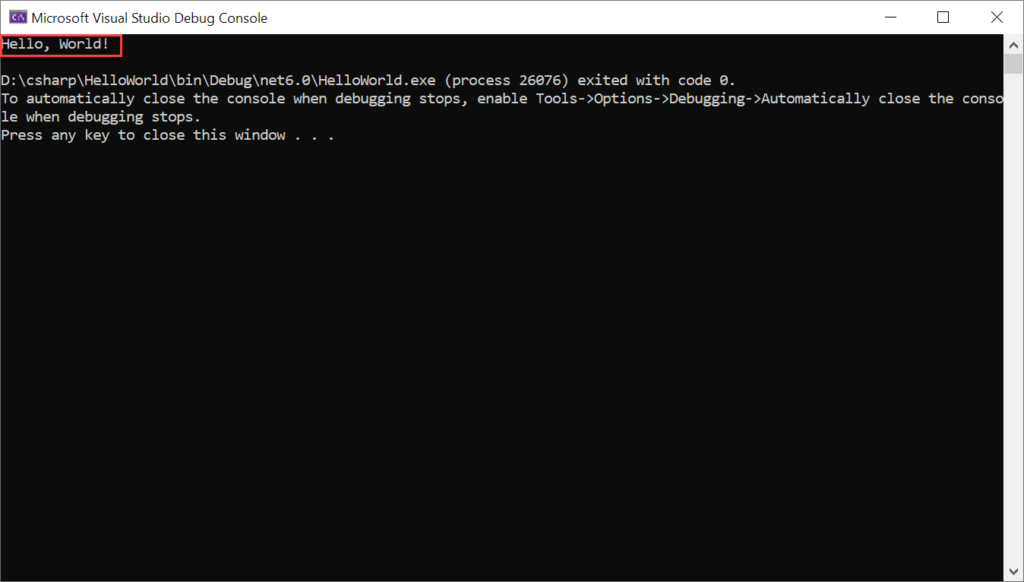
Congratulation! you have successfully created and run the Hello, World! program.
Using the using static syntax
C# 6 allows you to shorten the code by using the “using static
” syntax. So instead of using the Console.WriteLine
every time you write a string to the console:
Console.WriteLine("Hello, World!");
Code language: C# (cs)
You can use the using static
syntax like this:
using static System.Console;
WriteLine("Hello, World!");
Code language: JavaScript (javascript)
By doing this, you can use the WriteLine
method everywhere in the file without specifying the Console
.
Summary
- Use Visual Studio to create a Console App project for creating a command-line program that runs on .NET core on Windows, macOS, and Linux.
- Use
Console.WriteLine
method to write a string to the console window.