Summary: in this tutorial, you’ll learn how to delete data from the database tables in EF Core.
Setting up a sample project
First, download the HR sample project:
Download EF Core HR Sample Project
Second, run the Add-Migration
command in the Package Manager Console (PMC) to create a new migration:
Add-Migration Initial
Code language: C# (cs)
Third, execute the Update-Database
command in the PMC to create a new HR database and tables in the local SQL Server:
Update-Database
Code language: C# (cs)
Perform a simple delete
We’ll work with Departments
& Employees
tables:
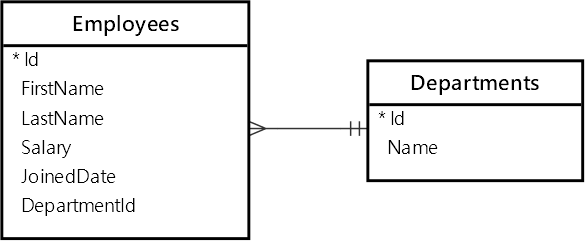
The following inserts a new department to the Departments
table:
using HR;
using var context = new HRContext();
var department = new Department() { Name = "Logistics" };
context.Departments.Add(department);
context.SaveChanges();
Code language: C# (cs)
The Departments
table will contain a row with Id 1:
Id Name
----------- ---------------
1 Logistics
Code language: plaintext (plaintext)
To delete a row from the database, you follow these steps:
- First, get the entity from the database.
- Second, remove the entity from the
by callingDbSet
‘sDbSet
Remove()
method. - Third, apply the change to the database by calling the
SaveChanges()
method.
For example, the following gets the department with Id 1 and removes it from the database:
using HR;
using var context = new HRContext();
// get the deparment
var department = context.Departments.Find(1);
if(department is not null)
{
// remove it
context.Departments.Remove(department);
// commit the change to the database
context.SaveChanges();
}
Code language: C# (cs)
How it works.
First, get the department with id 1 from the database:
var department = context.Departments.Find(1);
Code language: C# (cs)
EF Core executes a SELECT
statement that retrieves a department by id:
-- [Parameters=[@__p_0='1'], CommandType='Text', CommandTimeout='30']
SELECT TOP(1) [d].[Id], [d].[Name]
FROM [Departments] AS [d]
WHERE [d].[Id] = @__p_0
Code language: C# (cs)
Second, remove the department from the DbSet
and call the SaveChanges()
to remove the department from the database:
if(department is not null)
{
// remove it
context.Departments.Remove(department);
// commit the change to the database
context.SaveChanges();
}
Code language: C# (cs)
EF Core will perform a simple DELETE
statement that deletes the department with id 1 from the Departments table:
-- [Parameters=[@p0='1'], CommandType='Text', CommandTimeout='30']
SET IMPLICIT_TRANSACTIONS OFF;
SET NOCOUNT ON;
DELETE FROM [Departments]
OUTPUT 1
WHERE [Id] = @p0;
Code language: SQL (Structured Query Language) (sql)
Cascade Delete
The following program inserts an employee with a department into the Employees
and Departments
tables:
using HR;
using var context = new HRContext();
var employee = new Employee()
{
FirstName = "Jane",
LastName = "Doe",
Salary = 120_000,
JoinedDate = new DateTime(2023, 5, 1),
Department = new Department() { Name = "IT" }
};
context.Add(employee);
context.SaveChanges();
Code language: C# (cs)
The Employees
table (not all fields):
Id FirstName LastName DepartmentId
----------- --------------- --------------- ------------
1 Jane Doe 2
Code language: plaintext (plaintext)
The Departments
table:
Id Name
----------- ---------------
2 IT
Code language: plaintext (plaintext)
The DepartmentId
column of the Employees
table references the Id
column of the Employees
table. Due to the default foreign key constraint settings, when you delete a department, all employees that associate with the department will also be removed automatically by the database server. It’s called ON DELETE CASCADE
in the database.
For example:
using HR;
using var context = new HRContext();
// find department id 2
var department = context.Departments.Find(2);
if (department is not null)
{
// delete the department
context.Departments.Remove(department);
context.SaveChanges();
// all employees associcated with the department
// will be removed by the database
}
Code language: C# (cs)
If you view the data of the Departments
and Employees
tables, you’ll see that both tables are empty
Summary
- To delete an entity, first, get it from the database, then remove it from the
DbSet
, and call theSaveChanges()
to delete it from the database.