Summary: in this tutorial, you will learn how to sort data in EF Core using the OrderBy
, ThenBy
, OrderByDescending()
, and
methods.ThenBy
Descending()
We’ll use the Employee
entity that maps to the Employees
table in the database for the demonstration:
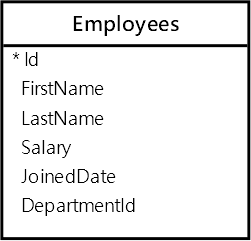
Sorting by one column in ascending order
To sort data by a column in ascending order, you use the LINQ
method. For example, the following uses the OrderBy
()OrderBy()
to sort the employees by first names in ascending order:
using HR;
using var context = new HRContext();
var list = context.Employees
.OrderBy(e => e.FirstName)
.ToList();
foreach (var e in list)
{
Console.WriteLine(e.FirstName);
}
Code language: C# (cs)
Output:
Abigail
Addison
Aiden
Alexander
Alexander
Amelia
...
Code language: plaintext (plaintext)
In this example, EF Core generates an SQL that selects data from the employee and uses the ORDER
BY
clause to sort the result set by the FirstName
column:
SELECT
[e].[Id],
[e].[DepartmentId],
[e].[FirstName],
[e].[JoinedDate],
[e].[LastName],
[e].[Salary]
FROM
[Employees] AS [e]
ORDER BY
[e].[FirstName]
Code language: SQL (Structured Query Language) (sql)
Sorting by two or more columns in ascending order
To sort the data by two columns, you use both the OrderBy
and
methods. For example, the following illustrates how to use the ThenBy()
and OrderBy
()
methods to sort employees by first names and last names:ThenBy()
using HR;
using var context = new HRContext();
var list = context.Employees
.OrderBy(e => e.FirstName)
.ThenBy(e => e.LastName)
.ToList();
foreach (var e in list)
{
Console.WriteLine($"{e.FirstName} {e.LastName}");
}
Code language: C# (cs)
Output:
Abigail Adams
Addison Hill
Aiden Wright
Alexander Green
Alexander Young
Amelia Scott
...
Code language: plaintext (plaintext)
Behind the scenes, EF Core generates an SQL statement that selects data from the Employees table and sorts the result set by the FirstName
and LastName
columns:
SELECT
[e].[Id],
[e].[DepartmentId],
[e].[FirstName],
[e].[JoinedDate],
[e].[LastName],
[e].[Salary]
FROM
[Employees] AS [e]
ORDER BY
[e].[FirstName],
[e].[LastName]
Code language: SQL (Structured Query Language) (sql)
Sorting by one column in descending order
To sort data in descending order, you use the LINQ
method. For example, the following uses the OrderByDescending() method to sort employees by their first names in descending order:OrderByDescending()
using HR;
using var context = new HRContext();
var list = context.Employees
.OrderByDescending(e => e.FirstName)
.ToList();
foreach (var e in list)
{
Console.WriteLine(e.FirstName);
}
Code language: C# (cs)
Output:
Zoe
Wyatt
William
Thomas
Sophia
Sophia
Sofia
...
Code language: plaintext (plaintext)
In this example, EF Core generates an SQL statement that uses the SELECT
to select data from the Employees table and ORDER
BY
DESC
to sort the employees by the first name in descending order:
SELECT
[e].[Id],
[e].[DepartmentId],
[e].[FirstName],
[e].[JoinedDate],
[e].[LastName],
[e].[Salary]
FROM
[Employees] AS [e]
ORDER BY
[e].[FirstName] DESC
Code language: SQL (Structured Query Language) (sql)
Sorting by two or more columns in descending order
To sort data by two or more columns in descending order, you use the LINQ OrderByDescending()
and ThenByDescending()
methods.
The following example uses the OrderByDescending()
and ThenByDescending()
methods to sort employees by first names and then by last names:
using HR;
using var context = new HRContext();
var list = context.Employees
.OrderByDescending(e => e.FirstName)
.ThenByDescending(e => e.LastName)
.ToList();
foreach (var e in list)
{
Console.WriteLine(e.FirstName);
}
Code language: C# (cs)
Output:
Zoe Hill
Wyatt Allen
William Taylor
Thomas Lopez
Sophia Wilson
Sophia Lee
Sofia Thomas
...
Code language: plaintext (plaintext)
EF Core executes an SQL that uses the SELECT
statement to select data from the Employees
tables and ORDER
BY
clause to sort data by FirstName
and LastName
in descending order:
SELECT
[e].[Id],
[e].[DepartmentId],
[e].[FirstName],
[e].[JoinedDate],
[e].[LastName],
[e].[Salary]
FROM
[Employees] AS [e]
ORDER BY
[e].[FirstName] DESC,
[e].[LastName] DESC
Code language: SQL (Structured Query Language) (sql)
Note that you can call the ThenByDescending()
method to sort the data by another column.
Sorting one column in ascending order and another column in descending order
The following uses the OrderBy()
and ThenByDescending()
methods to sort the employees by joined date in ascending order and then sort the sorted result set by first names in descending order:
using static System.Console;
using HR;
using var context = new HRContext();
var list = context.Employees
.OrderBy(e => e.JoinedDate)
.ThenByDescending(e => e.FirstName)
.ToList();
foreach (var e in list)
{
WriteLine($"{e.JoinedDate.ToShortDateString()} {e.LastName}");
}
Code language: C# (cs)
Output:
1/1/2023 Green
1/5/2023 Brown
1/15/2023 Doe
1/16/2023 Turner
1/29/2023 Wright
2/9/2023 Scott
...
Code language: plaintext (plaintext)
In this example, EF Core generates an SQL statement that sorts the JoinedDate
in ascending order and then the FirstName
in descending order:
SELECT
[e].[Id],
[e].[DepartmentId],
[e].[FirstName],
[e].[JoinedDate],
[e].[LastName],
[e].[Salary]
FROM
[Employees] AS [e]
ORDER BY
[e].[JoinedDate],
[e].[FirstName] DESC
Code language: SQL (Structured Query Language) (sql)
Summary
- Use
OrderBy()
method to sort data in ascending order. - Use
ThenBy()
to sort a sorted result set by another column in ascending order. - Use
OrderByDescending()
method to sort data in descending order. - Use
ThenByDescending()
method to sort a sorted result set by another column in descending order.